In Python, we deal with many types of Datatypes such as String, Integer, Boolean, Set, Tuple, List, Dictionary, Complex, and others. Python list, Set, Dictionary handle these all datatypes at the same time and in the same memory. This means the list, Set, Dictionary allows us to store all types of data and allows us to use it in the program. In order to use this Datatypes in the program, we need to manage it at some stage. list, Set, Dictionary have many methods, that help us to manage it very smoothly and without it any difficulty. We will learn some basic methods of the list.
Top Python Functions
abs( )
The abs() function in Python get the positive absolute value of a numerical value.
x = abs(-7.25)
print(x)
# output = 7.25
callable()
This function gets True, and if the passed object is callable, else it returns false.
def a():
b = 5
print(callable(a))
# output = True
chr()
Theis function gets the string character, which represents the defined unicode.
a = chr(87)
print(a)
# output: W
compile()
This function compiles the sources into a code.
a = compile('print(45)', 'test', 'eval')
exec(a)
# output: 45
delattr()
This function help us to remove the defined attribute from the declared object.
class User:
name = "Jaydon"
mob = 182182922
country = "Canada"
delattr(Person, 'age')
divmod()
It gets a tuple including the quotient and remainder when argument 1 is divided by argument 2.
a = divmod(6, 2)
print(a)
# output = (3, 0)
enumerate( )
This functions gets an enumerate object for a sequence or iterator.
a = ('john', 'ann', 'sia')
b = enumerate(a)
print(b)
# output = <enumerate object at 0x7f802a241a20>
filter()
It filters the sequence via a function, this function checks each elements in the iterator are true or not.
score = [15, 10, 20, 17, 26, 42]
def customFunc(a):
if a < 20:
return False
else:
return True
players = filter(customFunc, score)
for a in players:
print(a)
frozenset()
This function gets the unchangeable object.
list = ['Avengers', 'Wonder women', 'Batmen']
a = frozenset(list)
print(a)
getattr()
It gets the value of the defined attribute from the particular object.
class Student:
name = "Ann"
age = 28
country = "USA"
x = getattr(Student, 'age')
print(x)
output: 28
reduce()
This function iterates over each item in a list, or other iterable data type, and returns a single value.
from functools import reduce
def add_num(a, b):
return a+b
a = [1, 2, 3, 10]
print(reduce(add_num, a))
Output:16
split()
This function breaks a string based on set criteria. You can use it to count the number of words in a piece of text.
words = "column1 column2 column3"
words = words.split(" ")
print(words)
Output: ['column1', 'column2', 'column3']
enumerate()
It returns the length of an iterable and loops through its items simultaneously. Thus, while printing each item in an iterable data type, it simultaneously outputs its index.
fruits = ["grape", "apple", "mango"]
for i, j in enumerate(fruits):
print(i, j)
Output
0 grape
1 apple
2 mango
map()
This function lets you iterate over each item in an iterable. However, instead of producing a single result, it operates on each item independently.
You can perform mathematical operations on two or more lists using the this function.
b = [1, 3, 4, 6]
a = [1, 65, 7, 9]
def add(a, b):
return a+b
a = sum(map(add, b, a))
print(a)
Output: 96
range()
This function handy if you want to create a list of integers ranging between specific numbers without explicitly writing them out.
a = range(1, 6)
b = []
for i in a:
if i%2!=0:
b.append(i)
print(b)
Output:[1, 3, 5]
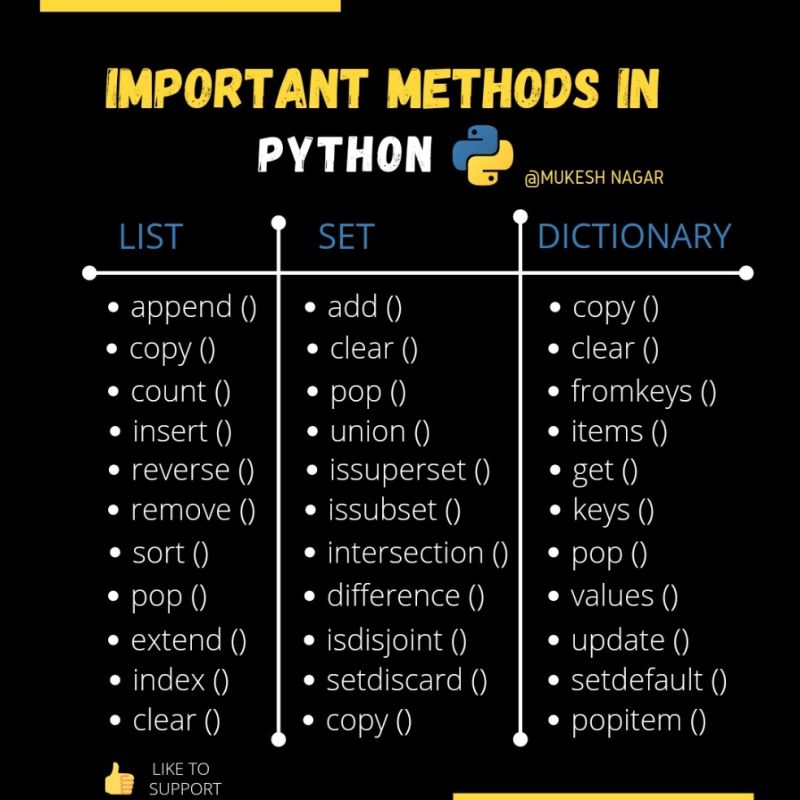
Recommended for you:
MOST COMMENTED
Tutorial
Important Methods in Matplotlib
Machine Learning
Bias and Variance Tradeoff Machine Learning
Tutorial
Multiclass and Multilabel Classification
Machine Learning
Reinforcement Learning in Machine Learning
Deep Learning
Alexnet Architecture Code
Machine Learning
Machine Learning Models Explained